Qualify and score your new users automatically with their email address
Use our API to identify high-value leads among your users. Send us a business email and we will reply with the JSON profile of the company as well as important information about the email.
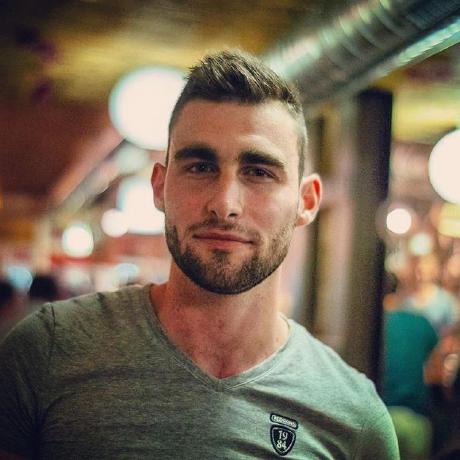
How it works
Many customers use our endpoint
to identify high-value leads among their users.For example, when someone registers on their website, they simply send the email address to our API, and we instantly return detailed information about the associated company.
Our customers also use our service to uncover important leads they may have missed in their database or CRM.
Scoring criteria
The scoring criteria will depend on your ideal customer profile (ICP). Below are key company data points to consider when qualifying your users.
You can assign a score when specific data points align with your ICP and notify your sales team via Slack to contact them.
We provide hundreds of datapoints for each company. You can browse the full list here.
Primary industry sector of the company.
hospitality
The total number of employees the company has.
200-500
Possible values
Revenue range of the company.
100m-200m
Possible values
Technologies used by the company.
API token
Before using The Companies API, you'll need an API token. Make sure to read our documentation to properly authenticate your requests.
Select or create a token
Code examples
Here are code examples in various languages to help you implement a minimal scoring system for your new users.
To go further, you might also find it useful to push high-value users to one of your Slack channels.
const scoreThresholds = {
highValue: 25,
employeePoints: 10,
revenuePoints: 20,
techStackPoints: 10
};
const validEmployeeRanges = ['50-200', '200-500', '500-1k', '1k-5k', '5k-10k', 'over-10k'];
const validRevenueRanges = ['10m-50m', '50m-100m', '100m-200m', '200m-1b', 'over-1b'];
const targetTechnologies = ['hubspot', 'salesforce', 'marketo'];
async function evaluateNewUser(email) {
try {
const domain = email.split('@')[1];
if (!domain) {
throw new Error('Invalid email format');
}
const response = await fetch(`https://api.thecompaniesapi.com/v2/companies/${domain}`, {
headers: {
Authorization: `Basic ${apiToken}`,
'Content-Type': 'application/json',
},
timeout: 5000,
});
if (!response.ok) {
throw new Error(`API request failed: ${response.status} ${response.statusText}`);
}
const { company, email: emailData } = await response.json();
if (emailData.isDisposable) {
return {
score: 0,
qualified: false,
reason: 'Disposable email address detected'
};
}
let score = 0;
if (validEmployeeRanges.includes(company.about.totalEmployees)) {
score += scoreThresholds.employeePoints;
}
if (validRevenueRanges.includes(company.finances.revenue)) {
score += scoreThresholds.revenuePoints;
}
const hasTargetTech = targetTechnologies.some(tech =>
company.technologies.active.includes(tech)
);
if (hasTargetTech) {
score += scoreThresholds.techStackPoints;
}
return {
score,
qualified: score >= scoreThresholds.highValue,
};
} catch (error) {
console.error('Error evaluating user:', error);
throw error;
}
}
Need help implementing your use case?
Reach out to us in the chat below, and let's solve it together!