How to build your company search engine
Learn how to create a powerful company search engine using our API. This guide covers endpoint usage, segmentation, and filtering techniques, complete with code examples and pricing details.
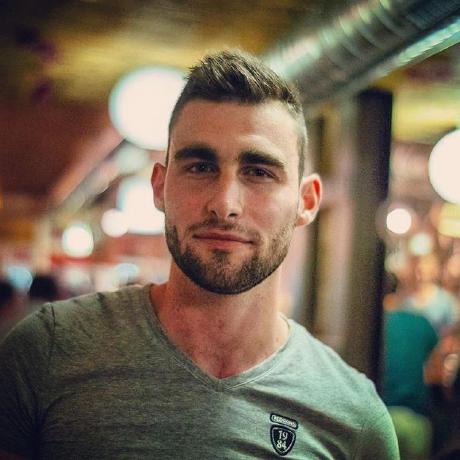
Introduction
Want to build a powerful company search engine like the one we offer? Our API makes it simple. In this guide, you'll learn:
- How to use the endpoint for powerful search capabilities.
- How our segmentation system works to filter our database.
- What company attributes you can search and filter by.
- Code examples in multiple languages to get you started.
- Pricing and usage details.
Cognizantcognizant.com | Over 10,000 employees | Over $1 billion | Public Company | Over 1 billion | United States North America | Teaneck Township Bergen County, New Jersey | 1994 | --- | --- | a month ago | High (105) | ||||
Fortinetfortinet.com | Over 10,000 employees | Over $1 billion | Public Company | 10M - 50M | United States North America | Sunnyvale Santa Clara County, California | 2000 | --- | --- | 3 months ago | High (99) | ||||
Microsoftmicrosoft.com | Over 10,000 employees | Over $1 billion | Public Company | Over 1 billion | United States North America | Redmond King County, Washington | 1975 | --- | 11 days ago | High (99) | |||||
Jabiljabil.com | Over 10,000 employees | Over $1 billion | Public Company | Over 1 billion | United States North America | Saint Petersburg Pinellas County, Florida | 1992 | --- | --- | a month ago | High (98) | ||||
Vestasvestas.com | Over 10,000 employees | Over $1 billion | Public Company | 10M - 50M | Denmark Europe | Aarhus Central Denmark Region | 1945 | --- | --- | a month ago | High (98) |
API token
Before using The Companies API, you'll need an API token. Make sure to read our documentation to properly authenticate your requests.
Select or create a token
How it works
Our company search endpoint
provides powerful search capabilities through a simpleGET
request. It returns a customizable number of matching companies based on your search criteria.You can also easily navigate between result pages, sort companies by any attribute and combine multiple filters together.
Each company returned in the full response costs 1 credit. You can browse our complete pricing plans to find the best option for your needs
Use the simplified=true
parameter to get a lightweight version of company data. Simplified responses are free and don't consume any credits. They are perfect for displaying search result previews or lists.
Our segmentation system
The query
parameter for the
- attribute: The attribute you want to filter by (e.g.,
about.industries
,about.totalEmployees
,finances.revenue
). Refer to the API endpoint documentation for a full list of available attributes. - operator: Logical operator to combine the values. Use
and
to require all values to be true, oror
to require at least one value to be true. - sign: The comparison sign for the condition. Options include:
equals
: Matches if the specified value is included in the attribute value.exactEquals
: Matches if the specified value exactly matches the attribute value.notEquals
: Matches if the specified value is not included in the attribute value.
- values: An array of values to match against the attribute (e.g.,
["saas", "software"]
for theabout.industries
attribute).
An example is worth a thousand words:
[
// Match companies in both SaaS AND software industries
{
"attribute": "about.industries",
"operator": "and",
"sign": "equals",
"values": ["saas", "software"]
},
// Match companies with revenue NOT in the 50m-100m range
{
"attribute": "finances.revenue",
"operator": "or",
"sign": "notEquals",
"values": ["50m-100m"]
}
]
This query
must be converted into a string and URL-encoded before being used in the query
parameter of the endpoint.
Code examples
Here are code examples in multiple languages to help you get started with building your own company search engine.
async function searchCompanies(query, page = 1, size = 25) {
const baseUrl = 'https://api.thecompaniesapi.com/v2/companies';
const params = new URLSearchParams({
query: JSON.stringify(query),
page,
size
});
const url = `${baseUrl}?${params.toString()}`;
const response = await fetch(url, {
headers: {
Authorization: `Basic ${process.env.YOUR_API_TOKEN}`,
},
});
if (!response.ok) {
throw new Error(`API request failed: ${response.status}`);
}
return await response.json();
}
// Retrieve SaaS companies with revenue between 50m and 100m
const query = [
{ "attribute": "about.industries", "operator": "and", "sign": "equals", "values": ["saas"] },
{ "attribute": "finances.revenue", "operator": "or", "sign": "notEquals", "values": ["50m-100m"] }
]
await searchCompanies(query)
Need help implementing your use case?
Reach out to us in the chat below, and let's solve it together!